💻 adminCommentView
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>관리자 코멘트 관리</title>
<link href="resources/css/adminCommentView.css" rel="stylesheet">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-beta/css/bootstrap.min.css">
<script src="https://code.jquery.com/jquery-3.2.1.slim.min.js"></script>
<!--
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.11.0/umd/popper.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-beta/js/bootstrap.min.js"></script>
<link rel="stylesheet" href="https://bootswatch.com/5/lux/bootstrap.css">
-->
</head>
<body>
<!-- 전체 영역 -->
<div class="wrap">
<!-- 플로팅 버튼 영역 -->
<jsp:include page="../common/floatingButton.jsp" />
<!-- 헤더 영역 -->
<jsp:include page="../common/header.jsp" />
<!-- 컨텐츠 영역 (개별 구현 구역) -->
<div id="content_container">
<table id="admin_profile">
<tr>
<td width="220"><img src="resources/image/user/profile/admin.png" width="170" height="170"></td>
<td width="380" style="font-size:50px; font-weight:900; color:white;">관리자</td>
</tr>
</table>
<!-- !!! 본인이 맡은 탭 div에 id="selected_tab" 붙어녛기 !!!-->
<div id="admin_mypage_navi">
<div><a href="admin_list.me">회원 관리</a></div>
<div><a href="">콘텐츠 관리</a></div>
<div id="selected_tab"><a href="commentList.ad">코멘트 관리</a></div>
<div><a href="">이용권 관리</a></div>
<div><a href="">신고 관리</a></div>
<div><a href="admin_stat.st">통계 관리</a></div>
</div>
<!-- 이곳부터 본인 화면 구현 -->
<div id="commentManagementArea">
<br><br>
<div id="selectUserMail">
<select id="selectCommentMenu">
<option>이메일</option>
<option>닉네임</option>
<option>콘텐츠명</option>
</select>
<input type="text" id="inputUserMail" placeholder="검색할 유저의 정보를 입력하세요"> <button type="button" id="selectButton">검색</button>
</div>
<br><br>
<div id="commentListAll">
<br>
<table id="commentTable">
<thead>
<tr id="comment_head" class="line">
<th class="comment_head1" width="5%">선택</th>
<th class="comment_head1" width="15%;">유저 이메일</th>
<th class="comment_head1" width="10%;">유저 닉네임</th>
<th class="comment_head1" width="20%;">콘텐츠명</th>
<th class="comment_head1" width="10%;">별점</th>
<th class="comment_head1" width="40%">코멘트 내용</th>
</tr>
</thead>
<tbody>
<c:forEach var="r" items="${ list }">
<tr class="personalComment">
<td><input type="checkbox" name="selectContent" id="selectContent"><label for="selectContent"></label></td>
<td id="userMail">${ r.userId }</td>
<td id="userNickname">${ r.userNickname }</td>
<td id="ContentName">
<c:choose>
<c:when test="${ not empty r.movieTitle }">
${ r.movieTitle }
</c:when>
<c:otherwise>
${ r.tvName }
</c:otherwise>
</c:choose>
</td>
<td id="reviewStar">★ ${ r.reviewStar }</td>
<td id="review_content">${ r.reviewContent }</td>
</tr>
</c:forEach>
<tr><th colspan="6" style="text-align: right;"><button type="button" class="btn btn-danger">삭제</button></th></tr>
</tbody>
</table>
<br><br>
<div id="pagingArea">
<c:choose>
<c:when test="${ pi.currentPage eq 1 }">
<button type="button" onclick="location.href='#';" disabled>«</button>
</c:when>
<c:otherwise>
<button type="button" onclick="location.href='commentList.ad?cpage=${ pi.currentPage - 1}';">«</button>
</c:otherwise>
</c:choose>
<c:forEach var="p" begin="${ pi.startPage }" end="${ pi.endPage }" step="1">
<button type="button" onclick="location.href='commentList.ad?cpage=${ p }';">${ p }</button>
</c:forEach>
<c:choose>
<c:when test="${ pi.currentPage eq pi.maxPage }">
<button type="button" onclick="location.href='#';" disabled>»</button>
</c:when>
<c:otherwise>
<button type="button" onclick="location.href='commentList.ad?cpage=${ pi.currentPage + 1}';">${ p }</button>
</c:otherwise>
</c:choose>
</div>
</div> <!-- commentListAll 영역 끝 -->
</div> <!-- 본인 구현 영역 끝 -->
</div> <!-- 컨텐츠 영역 끝 -->
<!-- 푸터 영역 -->
<jsp:include page="../common/footer.jsp" />
</div> <!-- 전체 영역 끝 -->
</body>
</html>
💻 ReviewController
package com.urfavoriteott.urfavoriteott.review.controller;
import java.util.ArrayList;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import com.urfavoriteott.urfavoriteott.common.model.vo.PageInfo;
import com.urfavoriteott.urfavoriteott.common.template.Pagination;
import com.urfavoriteott.urfavoriteott.review.model.service.ReviewService;
import com.urfavoriteott.urfavoriteott.review.model.vo.Review;
@Controller
public class ReviewController {
@Autowired
private ReviewService reviewService;
/**
* 관리자 페이지 코멘트 관리에서 사용할 페이징 바 - 작성자 : 수빈
* @param currentPage
* @return
*/
@RequestMapping(value="commentList.ad")
public String selectAdminCommentList(@RequestParam(value="cpage", defaultValue="1") int currentPage, Model model) {
int listCount = reviewService.selectAdminCommentListCount();
int pageLimit = 10;
int boardLimit = 10;
PageInfo pi = Pagination.getPageInfo(listCount, currentPage, pageLimit, boardLimit);
ArrayList<Review> list = reviewService.selectAdminCommentList(pi);
model.addAttribute("pi", pi);
model.addAttribute("list", list);
return "admin/adminCommentView";
}
}
💻 ReviewService
package com.urfavoriteott.urfavoriteott.review.model.service;
import java.util.ArrayList;
import com.urfavoriteott.urfavoriteott.common.model.vo.PageInfo;
import com.urfavoriteott.urfavoriteott.review.model.vo.Review;
public interface ReviewService {
/**
* 관리자 페이지 코멘트 관리를 위한 페이징바 - 작성자: 수빈
* @param reviewNo : 코멘트 번호
* @return
*/
int selectAdminCommentListCount();
/**
* 관리자 페이지에서 코멘트 관리를 위해 모든 코멘트 조회 (select) - 작성자: 수빈
* @param reviewNo : 코멘트 번호
* @return
*/
ArrayList<Review> selectAdminCommentList(PageInfo pi);
/**
* 관리자 페이지 신고관리를 위한 페이징바 - 작성자: 수빈
* @param reviewNo : 코멘트 번호
* @return
*/
int selectReportedCommentListCount();
/**
* 관리자 페이지에서 신 관리를 위해 모든 코멘트 조회 (select) - 작성자: 수빈
* @param reviewNo
* @return
*/
ArrayList<Review> selectReportedCommentList(int reviewNo);
/**
* 컨텐츠 하단에 띄울 본인의 별점과 코멘트 및 최신 코멘트 3개 (select) - 작성자: 수빈
* @param reviewNo : 코멘트 번호
* @param movieId : 코멘트가 달린 컨텐츠
* @param tvId : 코멘트가 달린 컨텐츠
* @return
*/
ArrayList<Review> selectCommentList(int reviewNo, int movieId, int tvId);
/**
* 컨텐츠에서 더보기 눌렀을 때 뜨는 해당 컨텐츠에 대한 전체 코멘트 (select) - 작성자: 수빈
* @param reviewNo : 코멘트 번호
* @param movieId : 코멘트가 달린 컨텐츠
* @param tvId : 코멘트가 달린 컨텐츠
* @return
*/
ArrayList<Review> selectCommentAll(int reviewNo, int movieId, int tvId);
/**
* 마이페이지에서 나의 코멘트 조회 (select) - 작성자 : 수빈
* @param reviewNo : 코멘트 번호
* @param userNo : 유저 번호(로그인한 사용자와 일치 비교)
* @return
*/
ArrayList<Review> selectMyComment(int reviewNo, int userNo);
/**
* 코멘트 작성 메소드 (insert) - 작성자: 수빈
* @param r : 사용자가 작성한 코멘트
* @return
*/
int insertReview(Review r);
/**
* 코멘트 수정 메소드 (update) - 작성자: 수빈
* @param reviewNo : 수정할 코멘트의 번호
* @return
*/
int updateReview(int reviewNo);
/**
* 코멘트 삭제 메소드 (update) - 작성자: 수빈
* @param reviewNo : 삭제할 코멘트의 번호
* @return
*/
int deleteReview(int reviewNo);
}
💻 ReviewServiceImpl
package com.urfavoriteott.urfavoriteott.review.model.service;
import java.util.ArrayList;
import org.mybatis.spring.SqlSessionTemplate;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.urfavoriteott.urfavoriteott.common.model.vo.PageInfo;
import com.urfavoriteott.urfavoriteott.review.model.dao.ReviewDao;
import com.urfavoriteott.urfavoriteott.review.model.vo.Review;
@Service
public class ReviewServiceImpl implements ReviewService {
@Autowired
private ReviewDao reviewDao;
@Autowired
private SqlSessionTemplate sqlSession;
@Override
public int selectAdminCommentListCount() {
return reviewDao.selectAdminCommentListCount(sqlSession);
}
@Override
public ArrayList<Review> selectAdminCommentList(PageInfo pi) {
return reviewDao.selectAdminCommentList(sqlSession, pi);
}
@Override
public int selectReportedCommentListCount() {
return 0;
}
@Override
public ArrayList<Review> selectReportedCommentList(int reviewNo) {
return null;
}
@Override
public ArrayList<Review> selectCommentList(int reviewNo, int movieId, int tvId) {
return null;
}
@Override
public ArrayList<Review> selectCommentAll(int reviewNo, int movieId, int tvId) {
return null;
}
@Override
public ArrayList<Review> selectMyComment(int reviewNo, int userNo) {
return null;
}
@Override
public int insertReview(Review r) {
return 0;
}
@Override
public int updateReview(int reviewNo) {
return 0;
}
@Override
public int deleteReview(int reviewNo) {
return 0;
}
}
💻 ReviewDao
package com.urfavoriteott.urfavoriteott.review.model.dao;
import java.util.ArrayList;
import org.apache.ibatis.session.RowBounds;
import org.mybatis.spring.SqlSessionTemplate;
import org.springframework.stereotype.Repository;
import com.urfavoriteott.urfavoriteott.common.model.vo.PageInfo;
import com.urfavoriteott.urfavoriteott.review.model.vo.Review;
@Repository
public class ReviewDao {
public int selectAdminCommentListCount(SqlSessionTemplate sqlSession) {
return sqlSession.selectOne("reviewMapper.selectAdminCommentListCount");
}
public ArrayList<Review> selectAdminCommentList(SqlSessionTemplate sqlSession, PageInfo pi) {
int limit = pi.getBoardLimit();
int offset = (pi.getCurrentPage() -1) * limit;
RowBounds rowBounds = new RowBounds(offset, limit);
return (ArrayList)sqlSession.selectList("reviewMapper.selectAdminCommentList", null, rowBounds);
}
}
💻 review-mapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "mybatis-3-mapper.dtd" >
<mapper namespace="reviewMapper">
<resultMap id="reviewResultSet" type="review">
<result column="REVIEW_NO" property="reviewNo" />
<result column="USER_NO" property="userNo" />
<result column="MOVIE_ID" property="movieId" />
<result column="MOVIE_TITLE" property="movieTitle" />
<result column="TV_ID" property="tvId" />
<result column="TV_NAME" property="tvName" />
<result column="REVIEW_STAR" property="reviewStar" />
<result column="REVIEW_CONTENT" property="reviewContent" />
<result column="REVIEW_REGISTER_DATE" property="reviewDate" />
<result column="REVIEW_STATUS" property="reviewStatus" />
<result column="USER_ID" property="userId" />
<result column="USER_NICKNAME" property="userNickname" />
</resultMap>
<select id="selectAdminCommentListCount" resultType="_int">
SELECT COUNT(*)
FROM REVIEW
WHERE REVIEW_STATUS = 'Y'
</select>
<select id="selectAdminCommentList" resultMap="reviewResultSet">
SELECT REVIEW_NO
, MB.USER_ID
, MB.USER_NICKNAME
, MV.TITLE AS "MOVIE_TITLE"
, TV.NAME AS "TV_NAME"
, TO_CHAR(REVIEW_STAR, 'FM0.0') AS "REVIEW_STAR"
, REVIEW_CONTENT
FROM REVIEW R
LEFT JOIN MEMBER MB USING(USER_NO)
LEFT JOIN MOVIE MV ON(R.MOVIE_ID = MV.MOVIE_ID)
LEFT JOIN TV ON (R.MOVIE_ID = TV.TV_ID)
WHERE REVIEW_STATUS = 'Y'
ORDER BY REVIEW_REGISTER_DATE DESC
</select>
</mapper>
💻 Review
package com.urfavoriteott.urfavoriteott.review.model.vo;
import java.sql.Date;
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.Setter;
import lombok.ToString;
@NoArgsConstructor
@AllArgsConstructor
@Setter
@Getter
@ToString
public class Review {
private int reviewNo; // REVIEW_NO NUMBER
private String reviewStatus; // REVIEW_STATUS CHAR(1 BYTE)
private String reviewStar; // REVIEW_STAR NUMBER
private String reviewContent; // REVIEW_CONTENT LONG
private Date reviewDate; // REVIEW_DATE DATE
private int userNo; // USER_NO NUMBER
private String userId; // 코멘트에 대한 리스트를 보여줄때 가지고 올 이메일
private String userNickname; // 코멘트에 대한 리스트를 보여줄때 가지고 올 닉네임
private int movieId; // MOVIE_ID
private String movieTitle; // movie 테이블에서 가지고 올 한글 제목
private int tvId; // TV_ID
private String tvName; // tv 테이블에서 가지고 올 한글 제목
}
💻 mybatis-config.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "mybatis-3-config.dtd" >
<configuration>
<!-- MyBatis가 구동될 때 알아야 하는 설정 -->
<settings>
<setting name="jdbcTypeForNull" value="NULL" />
</settings>
<!-- 클래스 별칭 -->
<typeAliases>
<typeAlias type="com.urfavoriteott.urfavoriteott.review.model.vo.Review" alias="review" />
</typeAliases>
<!-- mapper 등록 -->
<mappers>
<mapper resource="/mappers/review-mapper.xml" />
</mappers>
</configuration>
기특해서 돌아버려
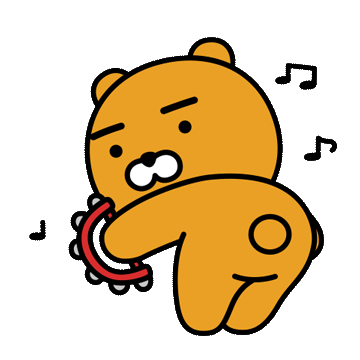